

So if we use std::mem::replace(&mut self.list, List::Nil) before destructuring, self.list will be replaced with a dummy value and we'll have ownership of the actual list, including both the value and the tail of the list.

The solution is to use std::mem::replace to get ownership not just of the data behind bx, but of the whole list. let val = *num is the same kind of "moving out of borrowed content" that we couldn't do before.
Rust namechanger windows#
Tip: You can also drag and drop files or folders from Windows Explorer to Advanced Renamer. Select the files you would like to rename and click Open. In the drop down list pick Files and a dialog for opening files appear. Click the Add menu item above the file list. u32 implements Copy so this isn't really a problem, but if you tried to make the linked list generic in the type of its elements, it simply wouldn't work. First you need to add some files to the list. That means we have to make a copy of it to return. The reason is that this value is still just a reference and we don't have owned access to the owner ( self.list).
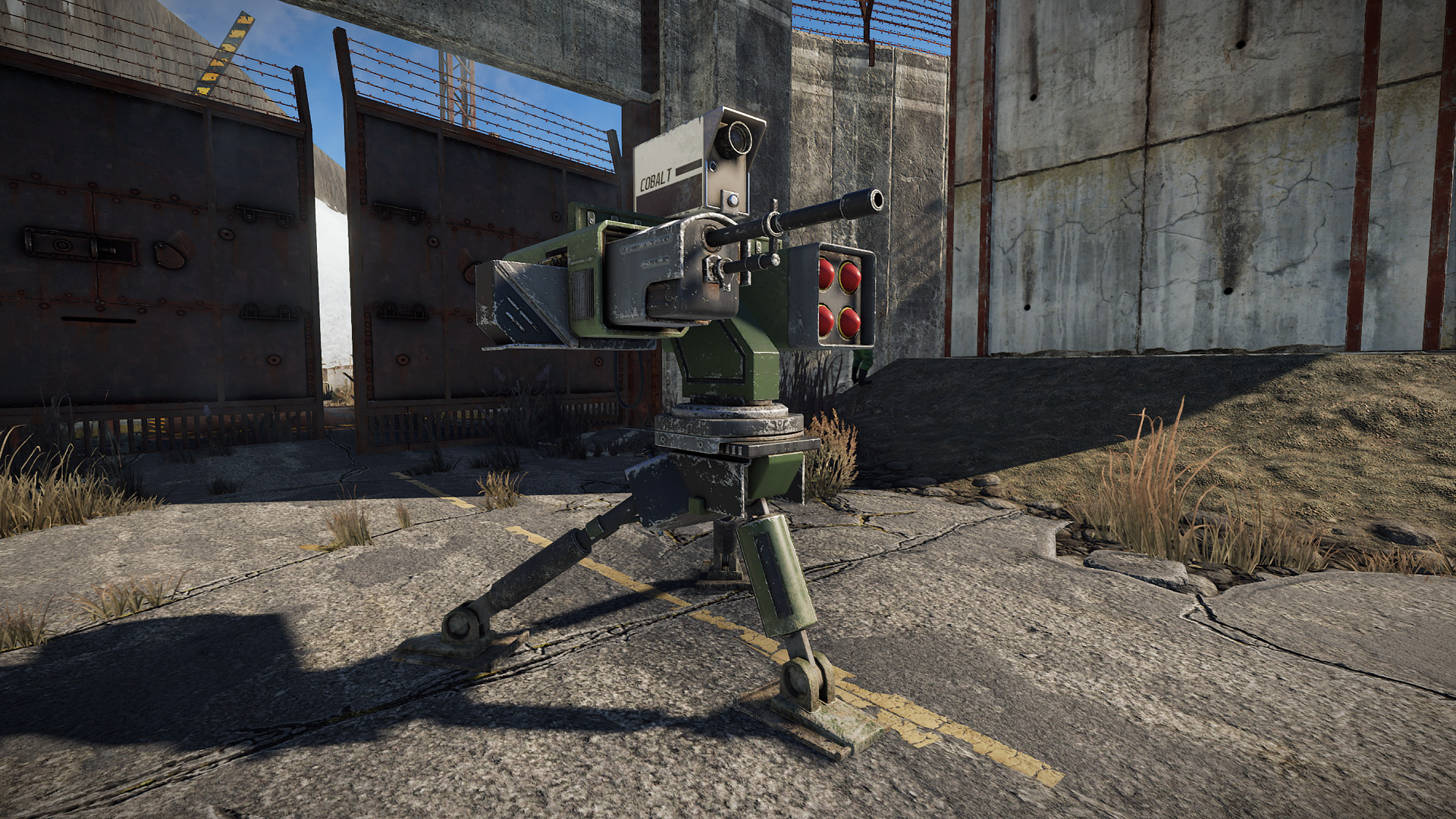
You may also be wondering about num and val and why we still have to make a temporary variable for that. Also, the into_iter call on list_inst is unnecessary since ListHolder already implements Iterator so it doesn't need to be turned into one. To be slightly more idiomatic, instead of &mut **bx, we could simply use bx.as_mut() to get a mutable reference from the box. Println!("", i) // Prints 1, 2, 3 as expected Self.list = *bx.clone() // This is the key line Is it possible or advisable to move the reference without copying it? # If I attempt to do this without bx.clone(), like so: self.list = **bx, I get "cannot move out of **bx which is behind a mutable reference." Which means that I need it to be owned, but I can't get an owned bx because I need to move it as a reference when I dereference it in the if let. I've been able to iterate over my List enum by copying the Box and assigning the list to the copied box, but intuitively, I feel like there must be a way to just "make it point to the next pointer in line". I'm practicing Rust concepts that I've learned while reading The Book.
